🍁transition 구현
타이머를 사용해 CSS에서 transition을 구현한 것처럼 자바스크립트에서도 비슷한 효과를 줄 수 있다.
자바스크립트는 제어를 할 수 있다는 점에서 CSS의 transiton과 차이가 있다.
[CSS] transition (전환)
🍁transition transition은 객체의 속성(상태) 값이 변화되는 과정을 시간 순서대로 보여주는 애니메이션 속성이다. 이 작업은 본래 JavaScript에서나 할 수 있는 것이었다. 하지만 지금은 CSS에서 간단하
isaac-christian.tistory.com
[CSS] animation (애니메이션)
🍁animation CSS 객체의 움직임을 단순하게 표현할 때에는 transition을 사용한다. animation은 그보다 더 나아가 움직임을 세밀하게 통제할 수 있는 옵션이 존재한다. [CSS] transition (전환) 🍁transition trans
isaac-christian.tistory.com
transition과 animation에 대해서는 위 글을 참고한다.
자바스크립트로 구현
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<style>
#box {
width: 200px;
height: 200px;
font-size: 20px;
background-color: darksalmon;
margin: 100px;
/* transition: all 1s; */
}
/* #box:hover {
transform: rotate(360deg);
} */
</style>
</head>
<body>
<div id="box">상자</div>
<script>
const box = document.getElementById('box');
let degree = 0;
let timer = 0;
box.addEventListener('click', function() {
if (timer == 0) {
timer = setInterval(function() {
box.style.transform = `rotate(${degree}deg)`;
degree++;
}, 10)
} else {
clearInterval(timer);
timer = 0;
}
});
</script>
</body>
</html>
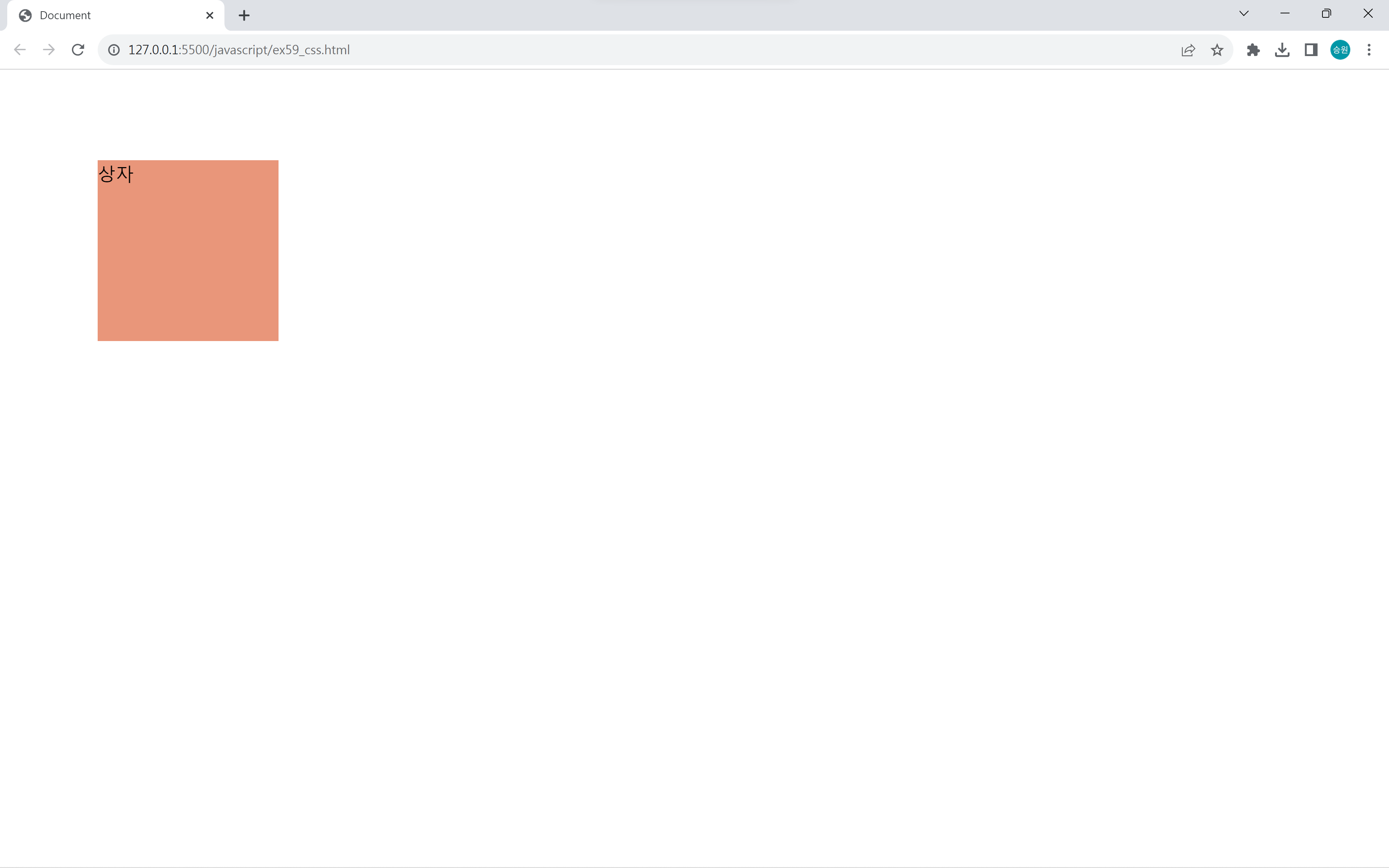
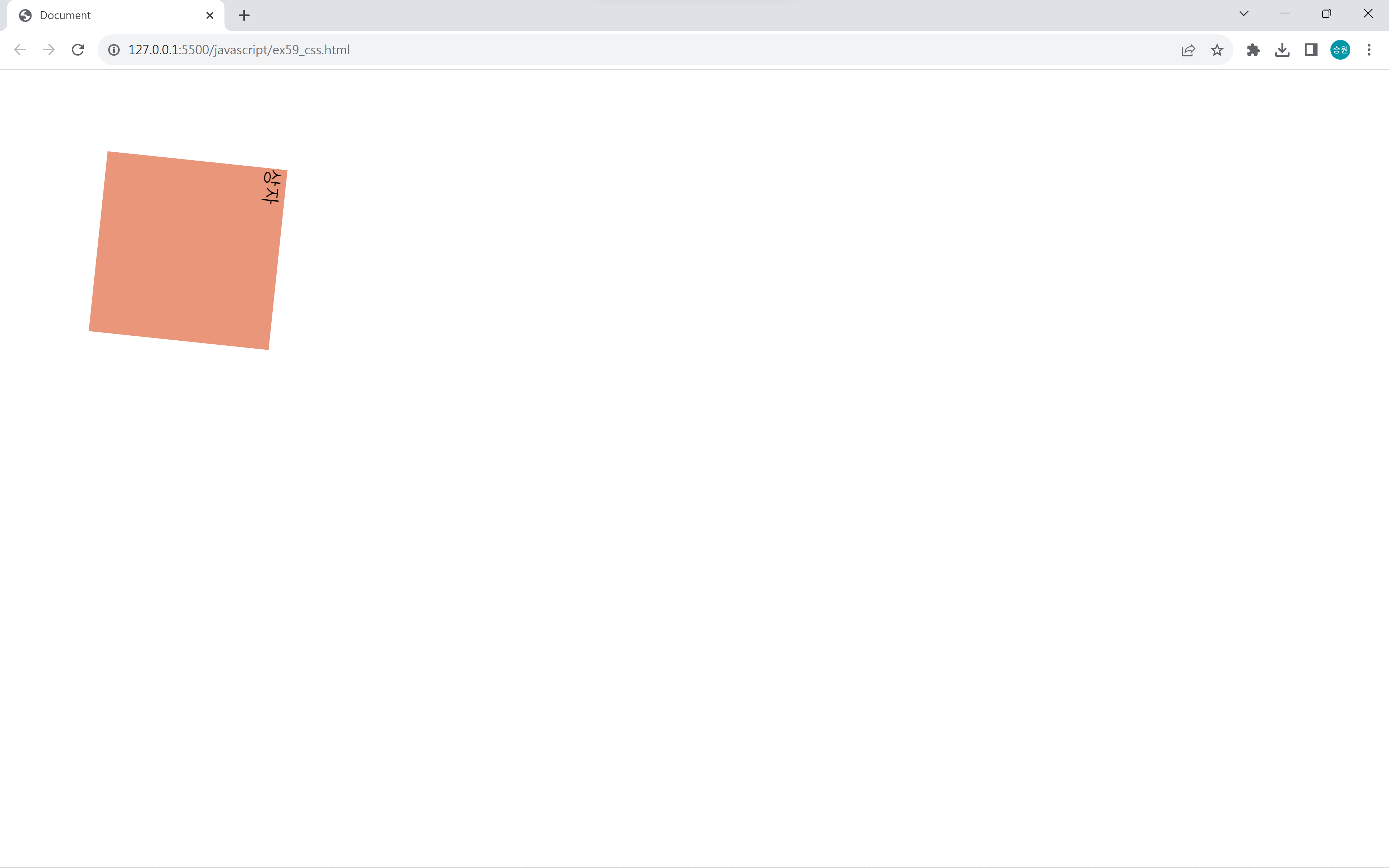
박스를 클릭하면 상자가 돌아가기 시작하고, 상자를 다시 클릭하면 돌아가던 상자가 멈춘다.
이런 식으로 자바스크립트는 개체에 대한 제어가 가능하다.
CSS와 자바스크립트로 구현
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<style>
#box {
width: 200px;
height: 200px;
font-size: 20px;
background-color: darksalmon;
margin: 100px;
transition: all 1s linear;
}
</style>
</head>
<body>
<div id="box">상자</div>
<script>
const box = document.getElementById('box');
let degree = 0;
let timer = 0;
box.addEventListener('click', function() {
box.style.transform = `rotate(360deg)`;
//box.style.transform = `rotate(${Math.random() * 3600}deg)`;
});
</script>
</body>
</html>
자바스크립트만 사용하여 단독으로 transition을 구현하지 않고, CSS를 혼합하여 사용하는 편이다.
이벤트와 속성에 대한 변화는 자바스크립트에서 부여하고, 타이머 역할은 CSS의 transition으로 구현한다.
즉, 순수한 타이머만으로 구현하지 말고 자바스크립트와 CSS를 적절하게 사용하도록 한다.
붙박이 메뉴
[CSS] transform (변형): 메뉴 구현
🍁transform transform에는 위치, 배율, 회전, 왜곡 변형까지 4가지 변형을 할 수 있다. translate, scale, rotate를 주로 사용한다. 기본 코드 상자1 상자2 상자3 .box { border: 1px solid black; width: 200px; height: 200px;
isaac-christian.tistory.com
자바스크립트를 추가해 위 글에서 구현한 붙박이 메뉴를 새롭게 구현해 보도록 하자.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<style>
#menu {
display: flex;
width: 630px;
position: fixed;
/* 수평정렬 set */
top: 0;
left: 0;
right: 0;
margin: 0 auto;
transform: translate(0px, -100px);
}
#menu img {
transition: all .5s;
}
/* 자바스크립트로 구현 */
/* #menu img:hover {
transform: translate(0px, 100px);
} */
</style>
</head>
<body>
<nav id="menu">
<img src="../asset/images/rect_icon01.png">
<img src="../asset/images/rect_icon02.png">
<img src="../asset/images/rect_icon03.png">
<img src="../asset/images/rect_icon04.png">
<img src="../asset/images/rect_icon05.png">
</nav>
<script>
const item=document.querySelectorAll('#menu > img');
let olditem;
for(let i=0; i<item.length; i++){
item[i].onclick=function(){
if(olditem!=null){ //메뉴 1개가 열려있다.
olditem.style.transform='translate(0px, 0px)';
}
if(olditem!=event.target){
event.target.style.transform='translate(0px, 90px)';
olditem=event.target;
}else{
event.target.style.transform='translate(0px, 0px)';
olditem=null;
}
}
}
</script>
</body>
</html>

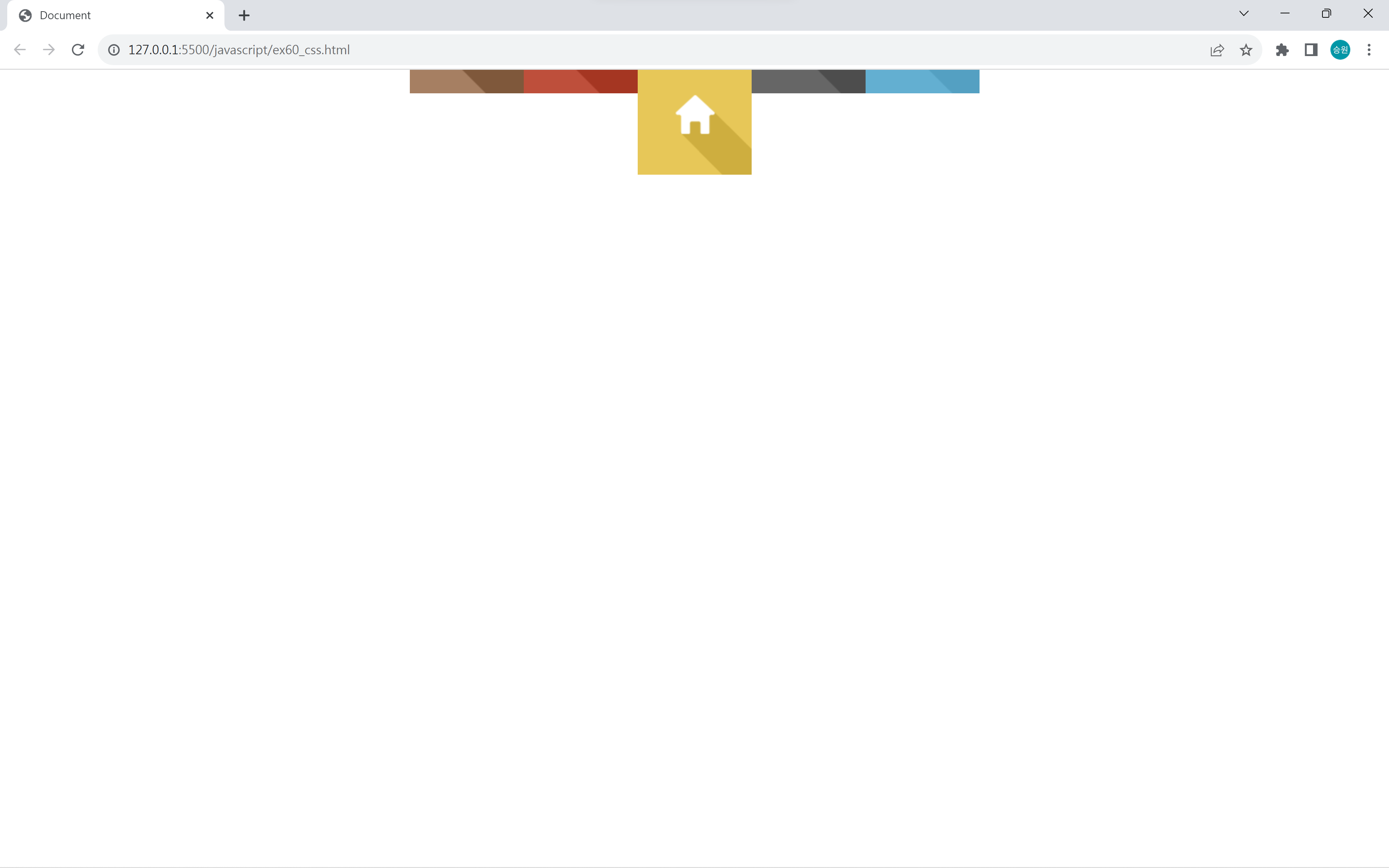
붙박이 메뉴를 클릭하면 해당 메뉴가 아래로 나타난다.
사진 슬라이더
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<style>
#box {
border: 10px solid cornflowerblue;
width: 250px;
height: 188px;
overflow: hidden;
}
#list {
/* border: 5px solid green; */
width: 1250px;
display: flex;
transition: all 1s;
}
/* 자바스크립트로 구현 */
/* #box:hover #list {
transform: translate(-250px, 0px);
} */
#sel1 {
font-size: 20px;
width: 250px;
height: 30px;
}
</style>
</head>
<body>
<h1>고양이</h1>
<div id="box">
<div id="list">
<img src="../asset/images/cat01.jpg">
<img src="../asset/images/cat02.jpg">
<img src="../asset/images/cat03.jpg">
<img src="../asset/images/cat04.jpg">
<img src="../asset/images/cat05.jpg">
</div>
</div>
<hr>
<select id="sel1">
<option value="0">고양이1</option>
<option value="1">고양이2</option>
<option value="2">고양이3</option>
<option value="3">고양이4</option>
<option value="4">고양이5</option>
</select>
<script>
const list = document.getElementById('list');
const sel1 = document.getElementById('sel1');
let dist = 0;
sel1.addEventListener('change', function() {
list.style.transform = `translate(-${sel1.value * 250}px, 0px)`;
})
window.onkeydown = function() {
list.style.transform = `translate(-${(event.keyCode - 49) * 250}px, 0px)`;
}
</script>
</body>
</html>
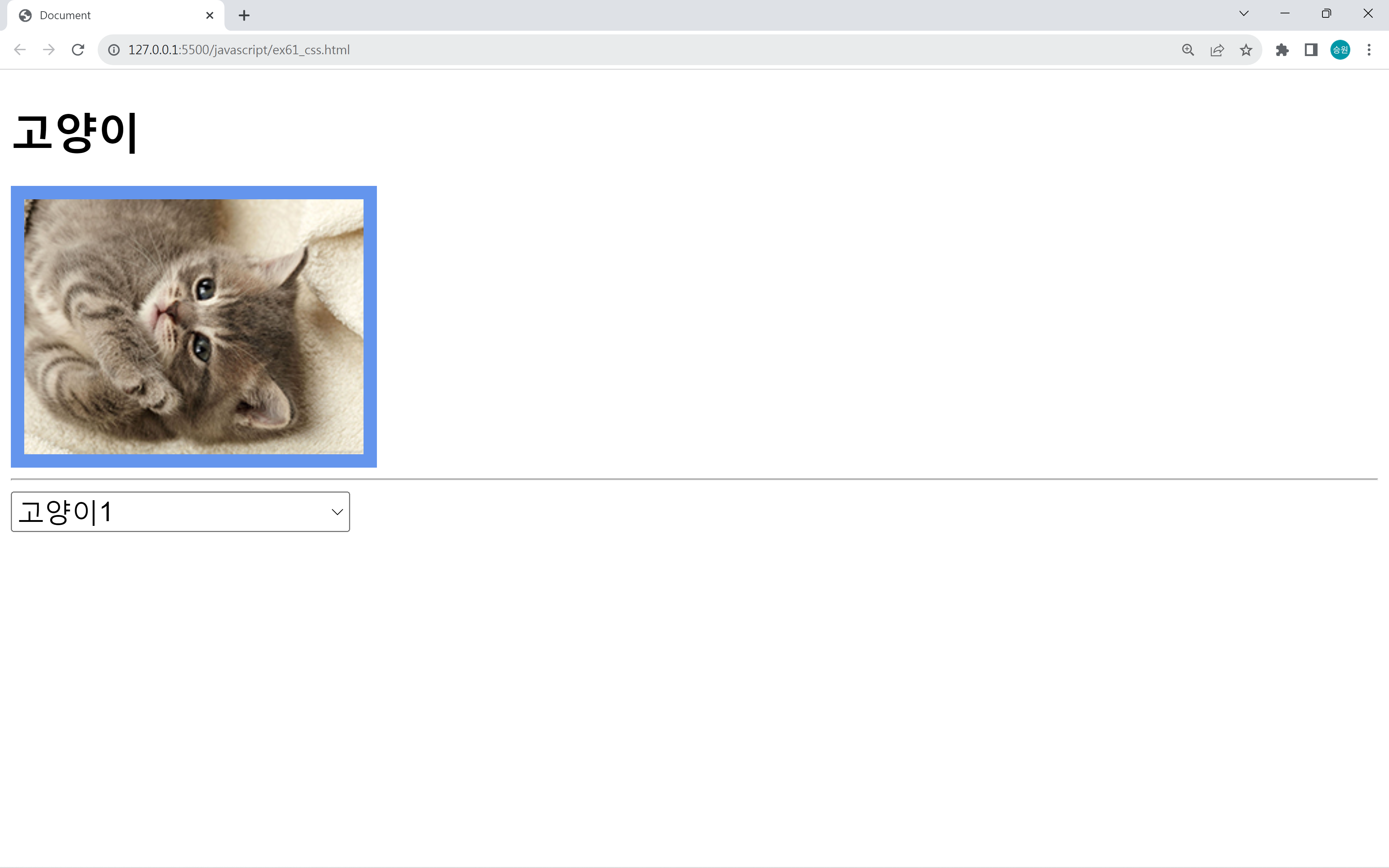
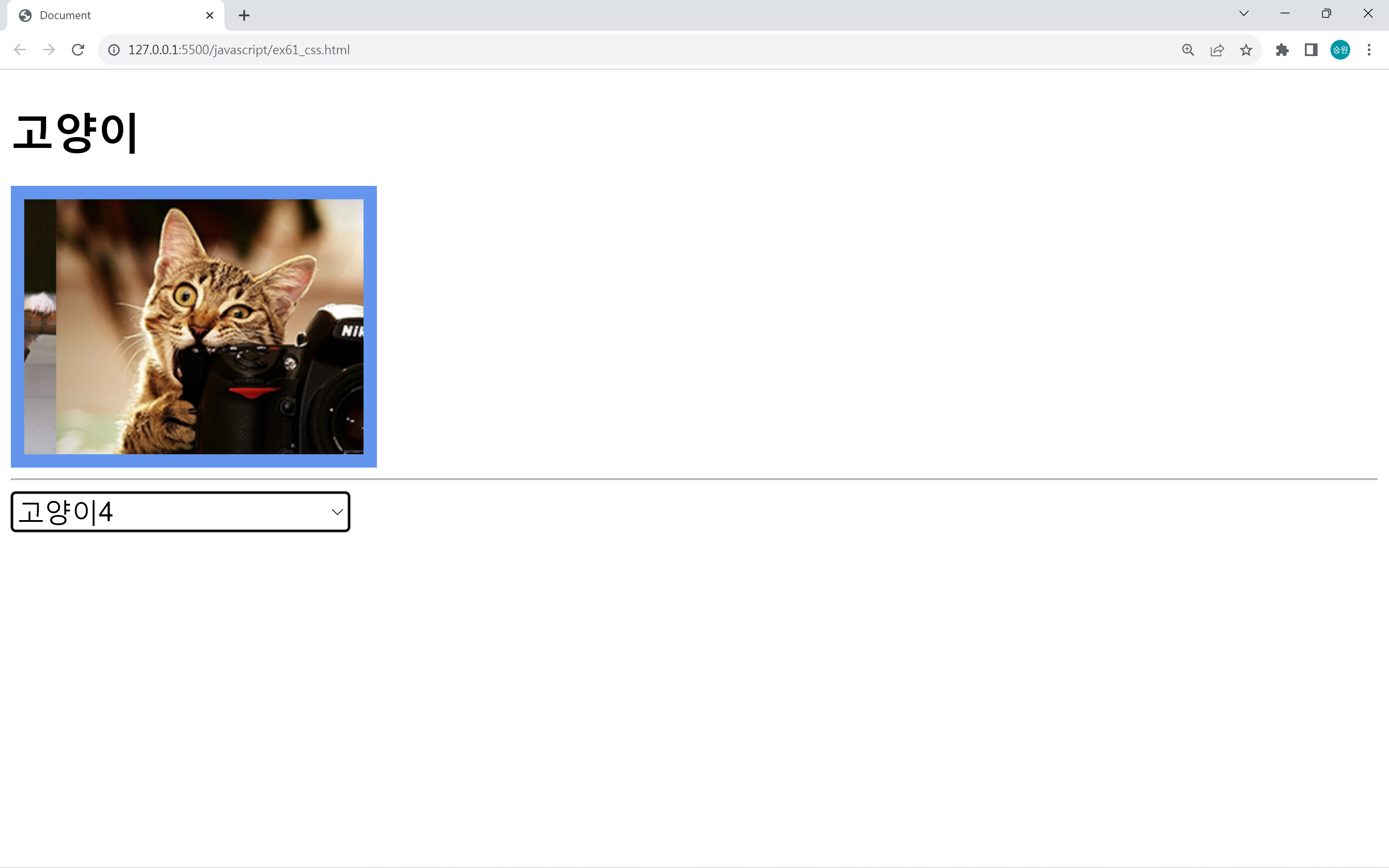
항목을 선택하거나 키보드 방향키를 입력하면 사진이 자연스럽게 다음으로 넘어간다.
배경 이미지 조작
하트
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<style>
#heart {
border: 1px solid black;
width: 300px;
height: 300px;
background-image: url(../asset/images/heart.png);
background-repeat: no-repeat;
}
</style>
</head>
<body>
<h1>배경 이미지 조작</h1>
<div id="heart"></div>
<hr>
<input type="button" value="두근 두근" id="btn1">
<script>
let x = 0;
document.getElementById('btn1').onclick = function() {
x -=300;
if (x < -600) {
x = 0;
}
document.getElementById('heart').style.backgroundPosition = x + 'px 0px';
}
</script>
</body>
</html>
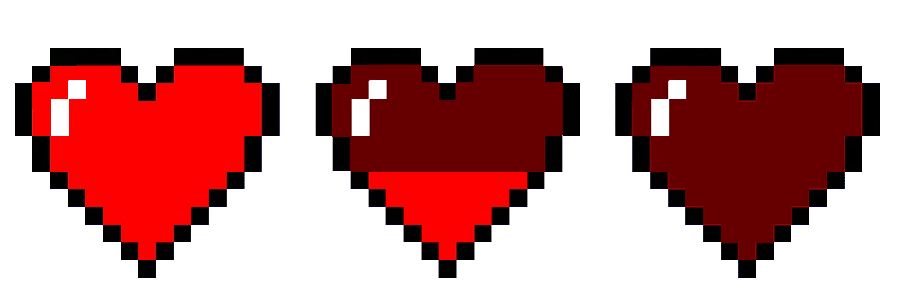
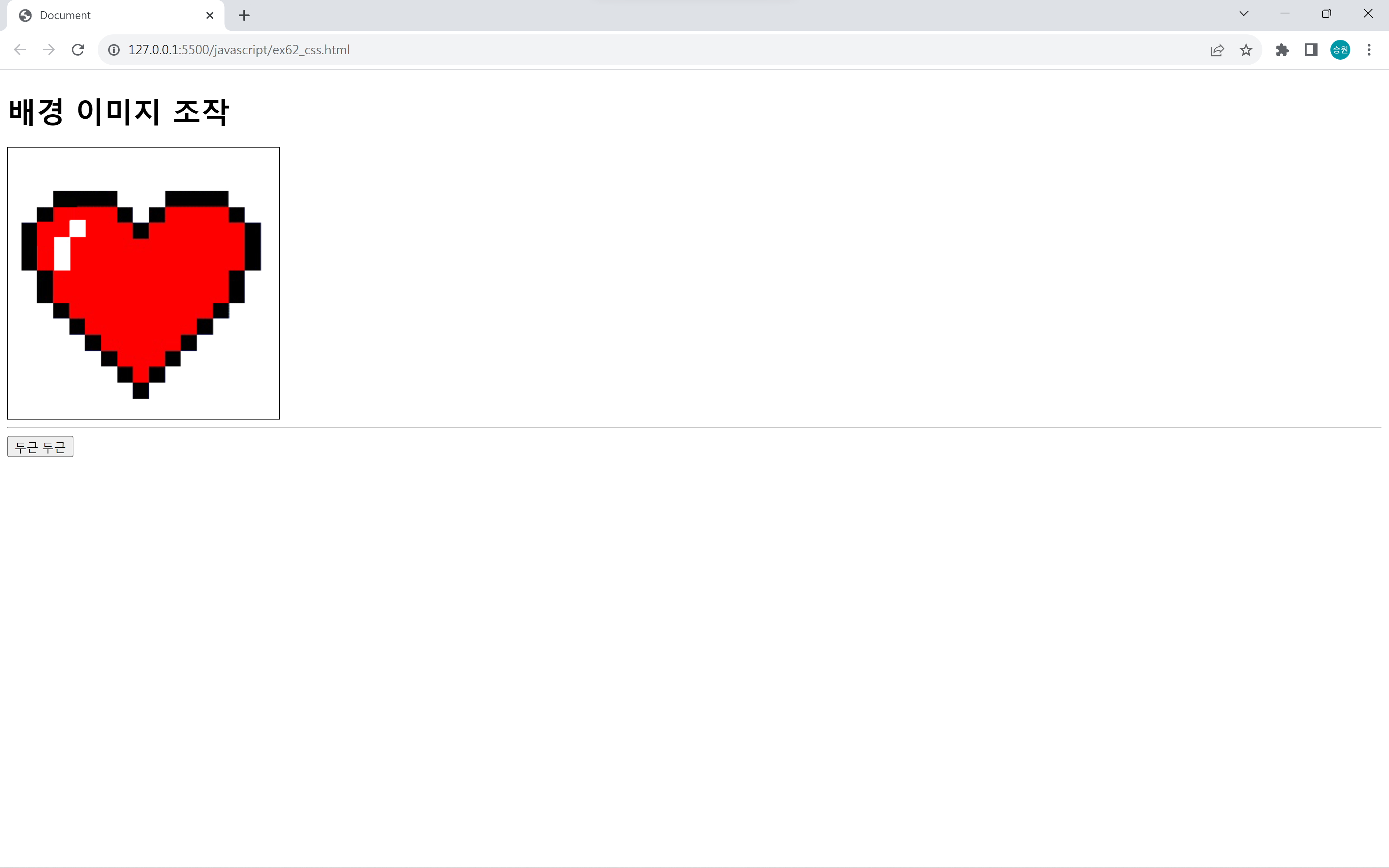
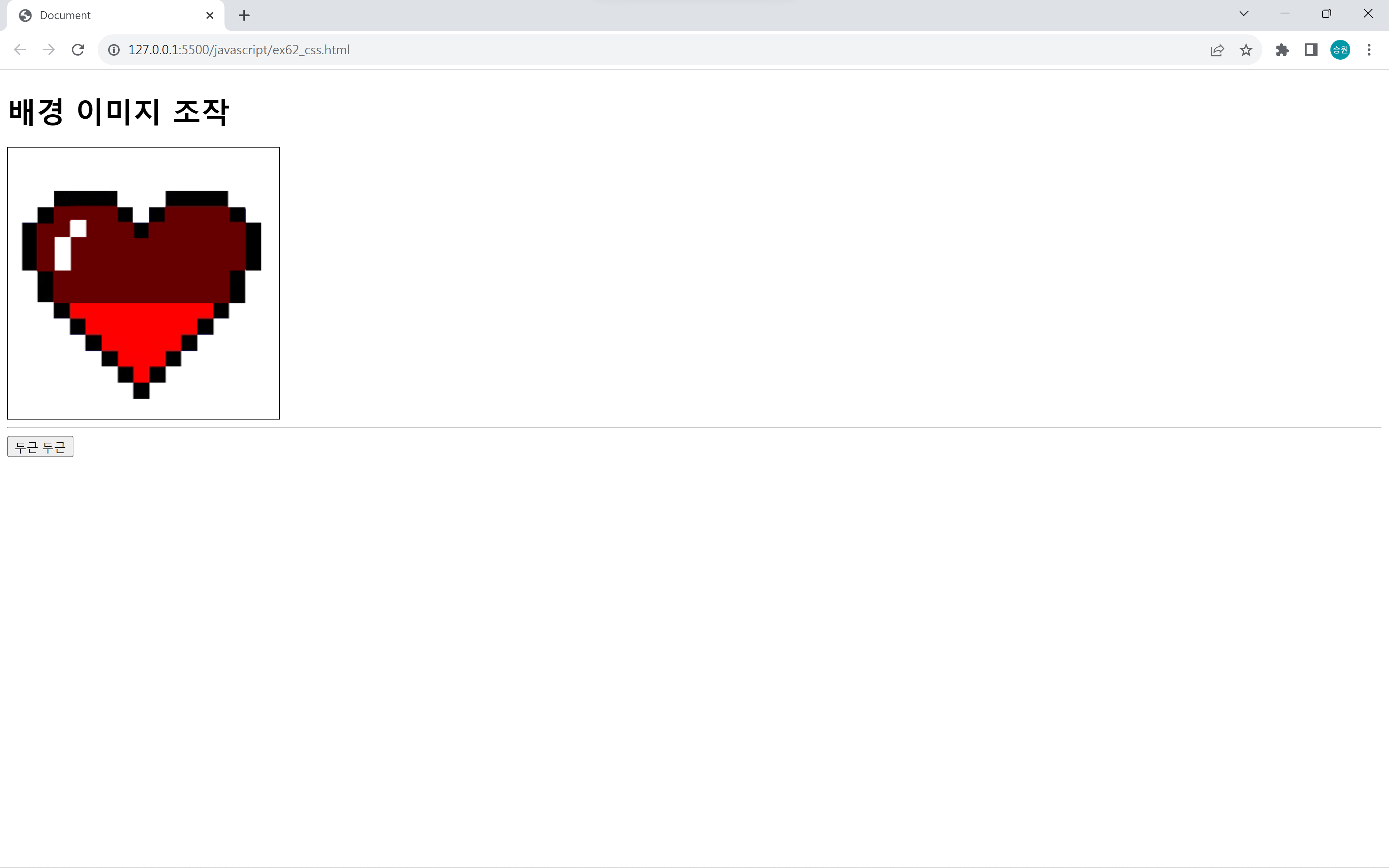
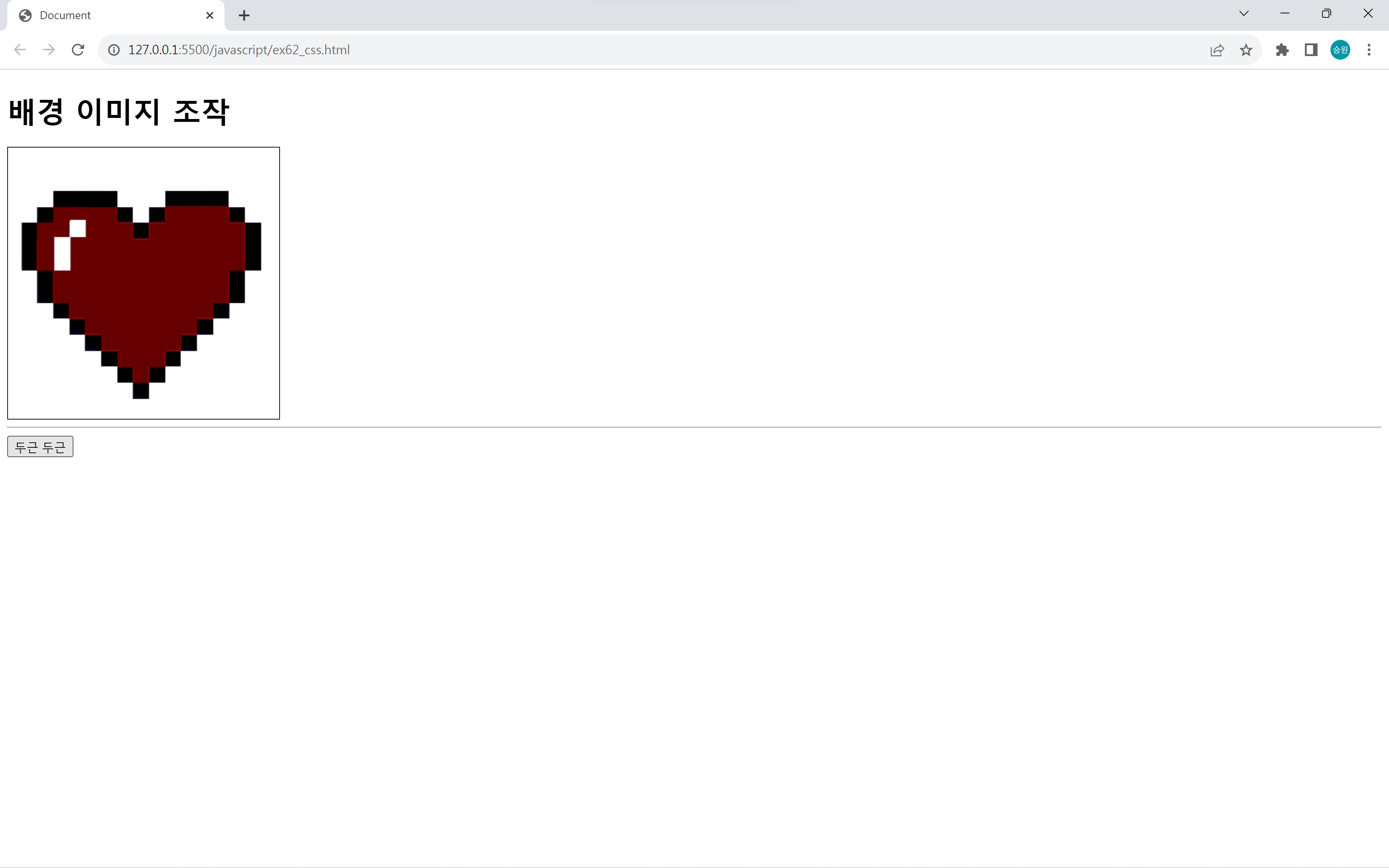
움직일 거리를 담당할 변수 x를 만들었다.
버튼을 클릭하면 하트 이미지가 전환된다.
퓨마
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<style>
#puma {
border: 1px solid black;
width: 510px;
height: 256px;
background-image: url(../asset/images/puma.png);
background-repeat: no-repeat;
}
</style>
</head>
<body>
<h1>퓨마</h1>
<div id="puma"></div>
<script>
const puma = document.getElementById('puma');
let x = 0;
let y = 0;
let timer = 0;
puma.onclick = function() {
if (timer == 0) {
timer = setInterval(function() {
x -= 512;
if (x < -(512 * 3)) {
x = 0;
y -= 256;
if (y < -256) {
y = 0;
}
}
puma.style.backgroundPosition = `${x}px ${y}px`;
}, 100);
} else {
clearInterval(timer);
timer = 0;
}
};
</script>
</body>
</html>

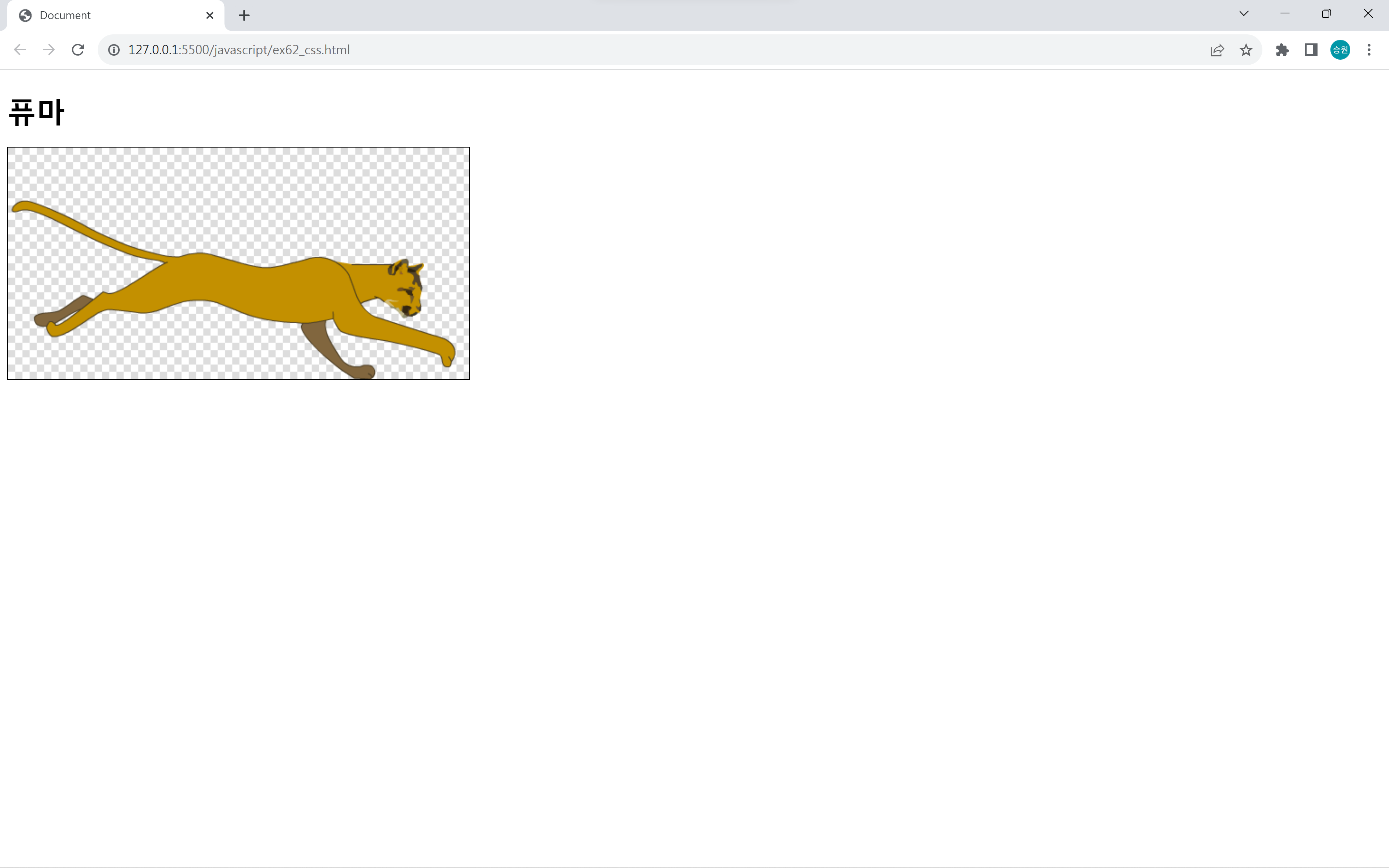
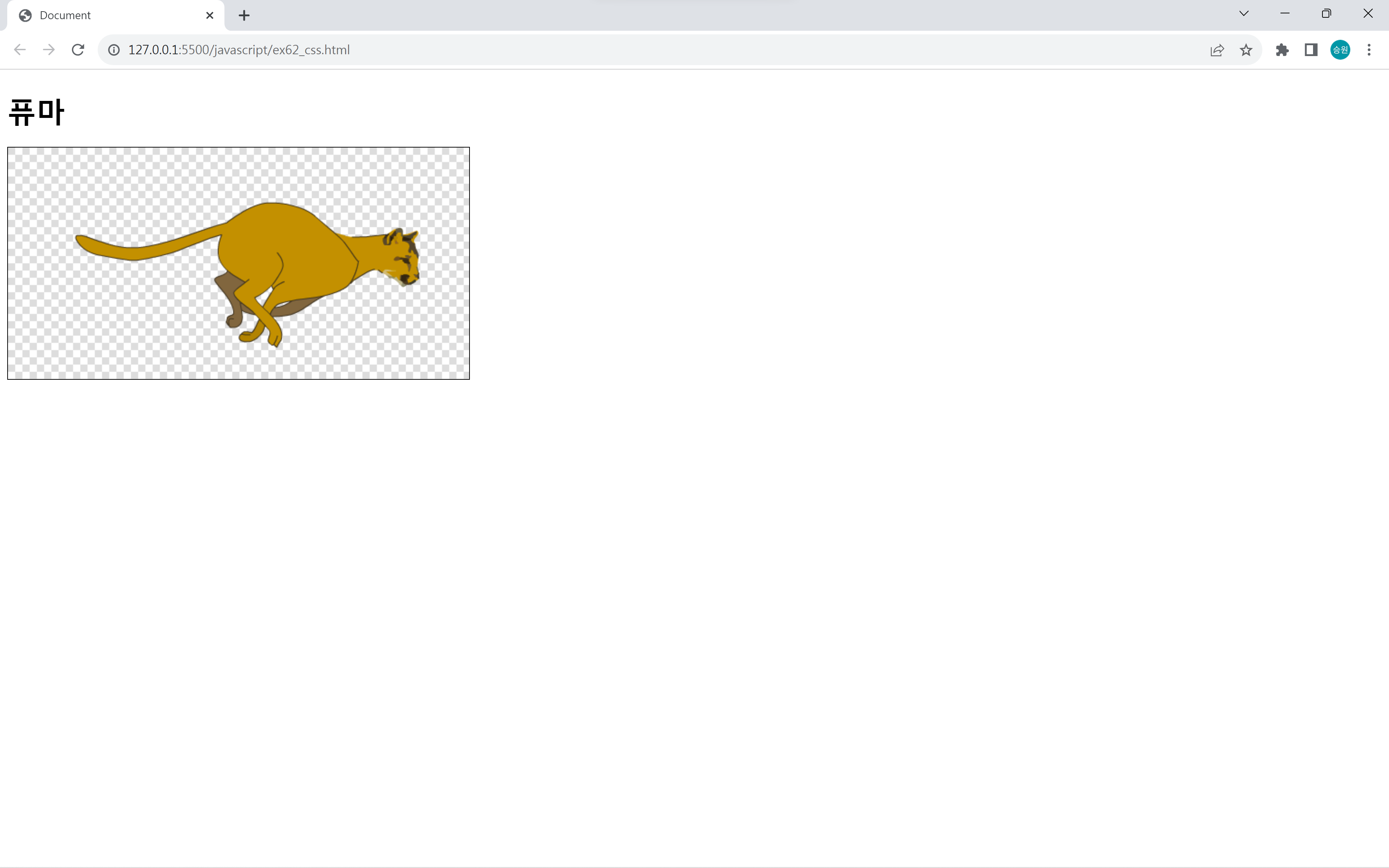
퓨마 이미지를 클릭하면 이미지가 순차적으로 전환하면서 퓨마가 뛰는 것처럼 보이게 된다.
🍁transition 구현
타이머를 사용해 CSS에서 transition을 구현한 것처럼 자바스크립트에서도 비슷한 효과를 줄 수 있다.
자바스크립트는 제어를 할 수 있다는 점에서 CSS의 transiton과 차이가 있다.
[CSS] transition (전환)
🍁transition transition은 객체의 속성(상태) 값이 변화되는 과정을 시간 순서대로 보여주는 애니메이션 속성이다. 이 작업은 본래 JavaScript에서나 할 수 있는 것이었다. 하지만 지금은 CSS에서 간단하
isaac-christian.tistory.com
[CSS] animation (애니메이션)
🍁animation CSS 객체의 움직임을 단순하게 표현할 때에는 transition을 사용한다. animation은 그보다 더 나아가 움직임을 세밀하게 통제할 수 있는 옵션이 존재한다. [CSS] transition (전환) 🍁transition trans
isaac-christian.tistory.com
transition과 animation에 대해서는 위 글을 참고한다.
자바스크립트로 구현
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<style>
#box {
width: 200px;
height: 200px;
font-size: 20px;
background-color: darksalmon;
margin: 100px;
/* transition: all 1s; */
}
/* #box:hover {
transform: rotate(360deg);
} */
</style>
</head>
<body>
<div id="box">상자</div>
<script>
const box = document.getElementById('box');
let degree = 0;
let timer = 0;
box.addEventListener('click', function() {
if (timer == 0) {
timer = setInterval(function() {
box.style.transform = `rotate(${degree}deg)`;
degree++;
}, 10)
} else {
clearInterval(timer);
timer = 0;
}
});
</script>
</body>
</html>
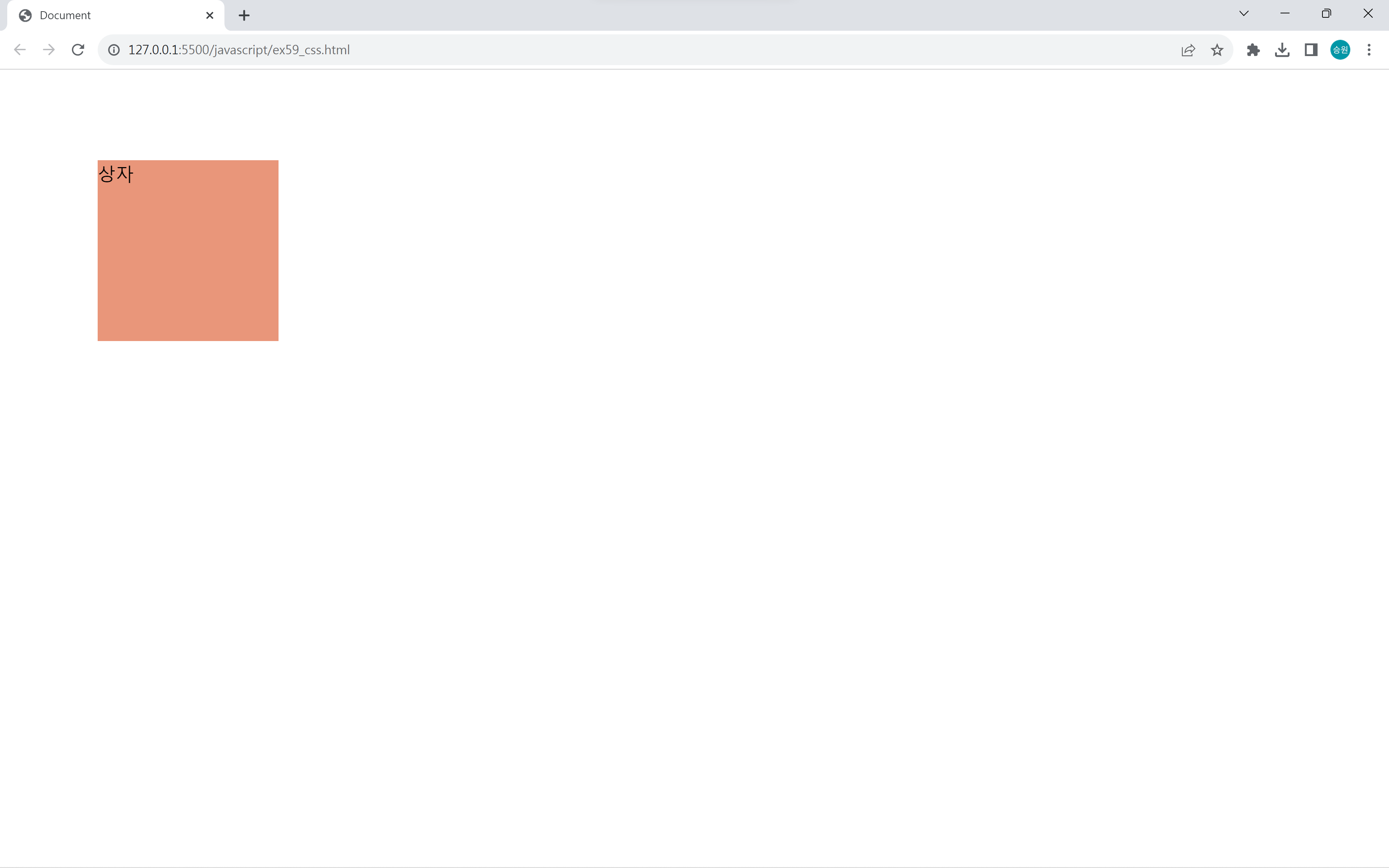
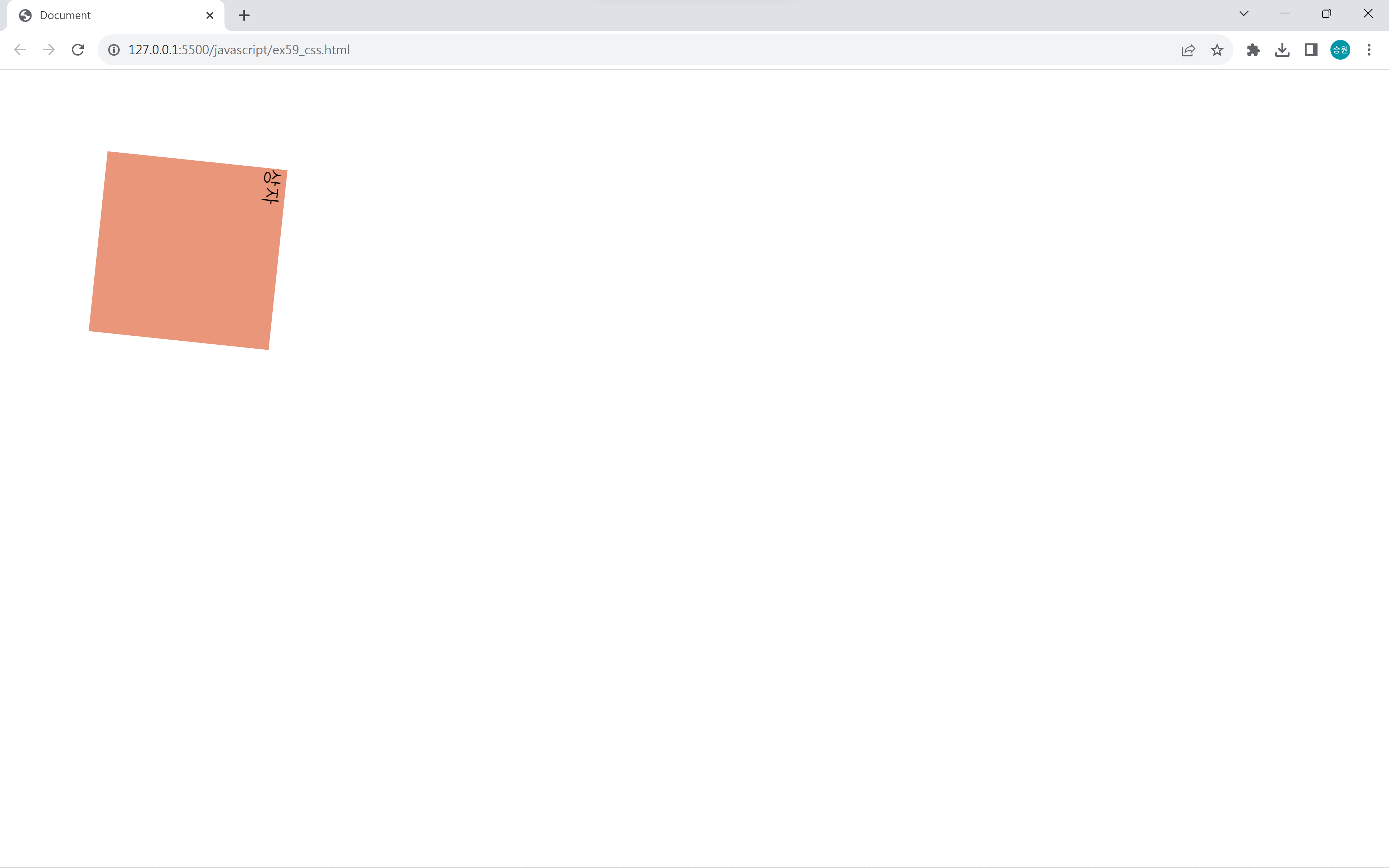
박스를 클릭하면 상자가 돌아가기 시작하고, 상자를 다시 클릭하면 돌아가던 상자가 멈춘다.
이런 식으로 자바스크립트는 개체에 대한 제어가 가능하다.
CSS와 자바스크립트로 구현
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<style>
#box {
width: 200px;
height: 200px;
font-size: 20px;
background-color: darksalmon;
margin: 100px;
transition: all 1s linear;
}
</style>
</head>
<body>
<div id="box">상자</div>
<script>
const box = document.getElementById('box');
let degree = 0;
let timer = 0;
box.addEventListener('click', function() {
box.style.transform = `rotate(360deg)`;
//box.style.transform = `rotate(${Math.random() * 3600}deg)`;
});
</script>
</body>
</html>
자바스크립트만 사용하여 단독으로 transition을 구현하지 않고, CSS를 혼합하여 사용하는 편이다.
이벤트와 속성에 대한 변화는 자바스크립트에서 부여하고, 타이머 역할은 CSS의 transition으로 구현한다.
즉, 순수한 타이머만으로 구현하지 말고 자바스크립트와 CSS를 적절하게 사용하도록 한다.
붙박이 메뉴
[CSS] transform (변형): 메뉴 구현
🍁transform transform에는 위치, 배율, 회전, 왜곡 변형까지 4가지 변형을 할 수 있다. translate, scale, rotate를 주로 사용한다. 기본 코드 상자1 상자2 상자3 .box { border: 1px solid black; width: 200px; height: 200px;
isaac-christian.tistory.com
자바스크립트를 추가해 위 글에서 구현한 붙박이 메뉴를 새롭게 구현해 보도록 하자.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<style>
#menu {
display: flex;
width: 630px;
position: fixed;
/* 수평정렬 set */
top: 0;
left: 0;
right: 0;
margin: 0 auto;
transform: translate(0px, -100px);
}
#menu img {
transition: all .5s;
}
/* 자바스크립트로 구현 */
/* #menu img:hover {
transform: translate(0px, 100px);
} */
</style>
</head>
<body>
<nav id="menu">
<img src="../asset/images/rect_icon01.png">
<img src="../asset/images/rect_icon02.png">
<img src="../asset/images/rect_icon03.png">
<img src="../asset/images/rect_icon04.png">
<img src="../asset/images/rect_icon05.png">
</nav>
<script>
const item=document.querySelectorAll('#menu > img');
let olditem;
for(let i=0; i<item.length; i++){
item[i].onclick=function(){
if(olditem!=null){ //메뉴 1개가 열려있다.
olditem.style.transform='translate(0px, 0px)';
}
if(olditem!=event.target){
event.target.style.transform='translate(0px, 90px)';
olditem=event.target;
}else{
event.target.style.transform='translate(0px, 0px)';
olditem=null;
}
}
}
</script>
</body>
</html>

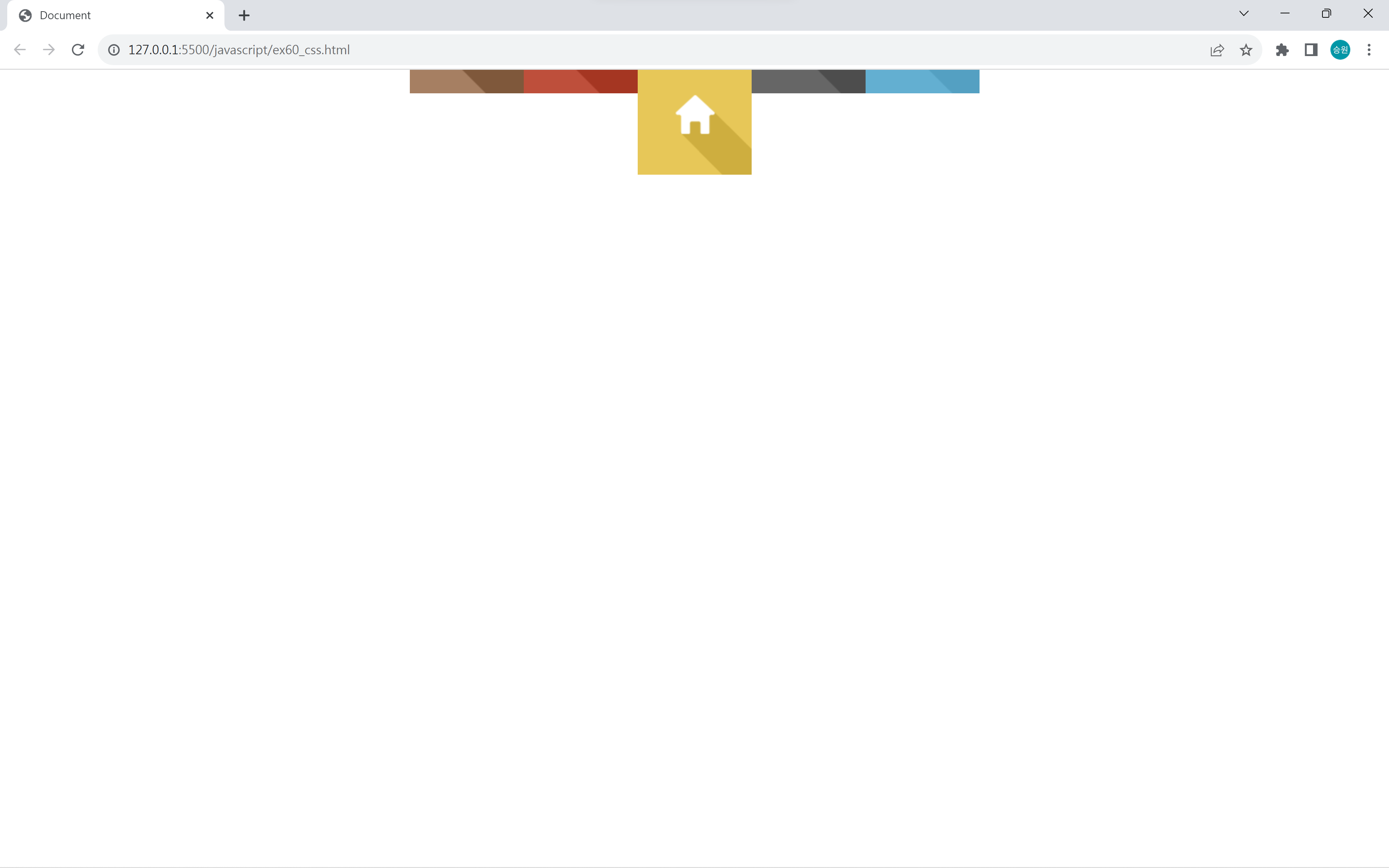
붙박이 메뉴를 클릭하면 해당 메뉴가 아래로 나타난다.
사진 슬라이더
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<style>
#box {
border: 10px solid cornflowerblue;
width: 250px;
height: 188px;
overflow: hidden;
}
#list {
/* border: 5px solid green; */
width: 1250px;
display: flex;
transition: all 1s;
}
/* 자바스크립트로 구현 */
/* #box:hover #list {
transform: translate(-250px, 0px);
} */
#sel1 {
font-size: 20px;
width: 250px;
height: 30px;
}
</style>
</head>
<body>
<h1>고양이</h1>
<div id="box">
<div id="list">
<img src="../asset/images/cat01.jpg">
<img src="../asset/images/cat02.jpg">
<img src="../asset/images/cat03.jpg">
<img src="../asset/images/cat04.jpg">
<img src="../asset/images/cat05.jpg">
</div>
</div>
<hr>
<select id="sel1">
<option value="0">고양이1</option>
<option value="1">고양이2</option>
<option value="2">고양이3</option>
<option value="3">고양이4</option>
<option value="4">고양이5</option>
</select>
<script>
const list = document.getElementById('list');
const sel1 = document.getElementById('sel1');
let dist = 0;
sel1.addEventListener('change', function() {
list.style.transform = `translate(-${sel1.value * 250}px, 0px)`;
})
window.onkeydown = function() {
list.style.transform = `translate(-${(event.keyCode - 49) * 250}px, 0px)`;
}
</script>
</body>
</html>
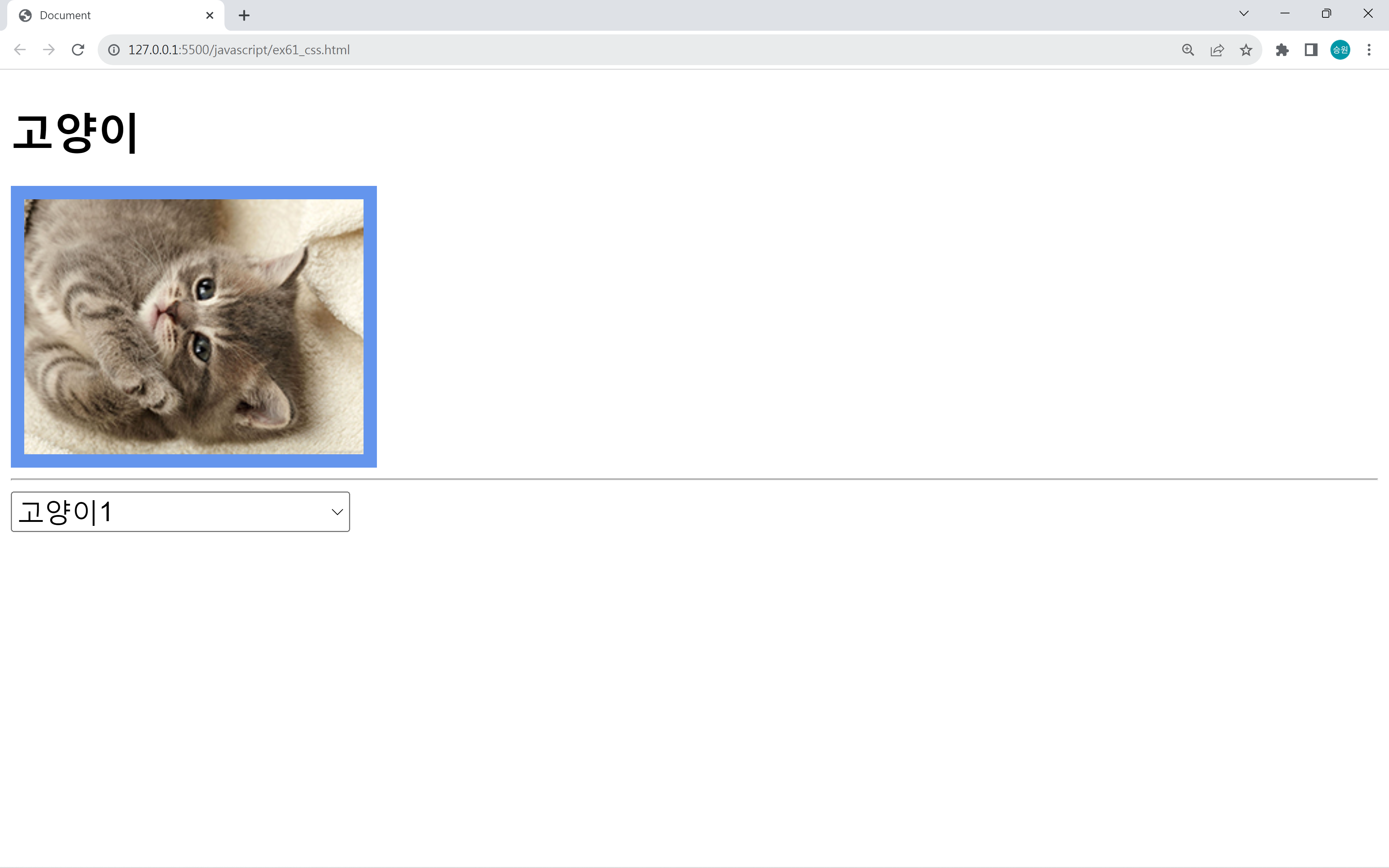
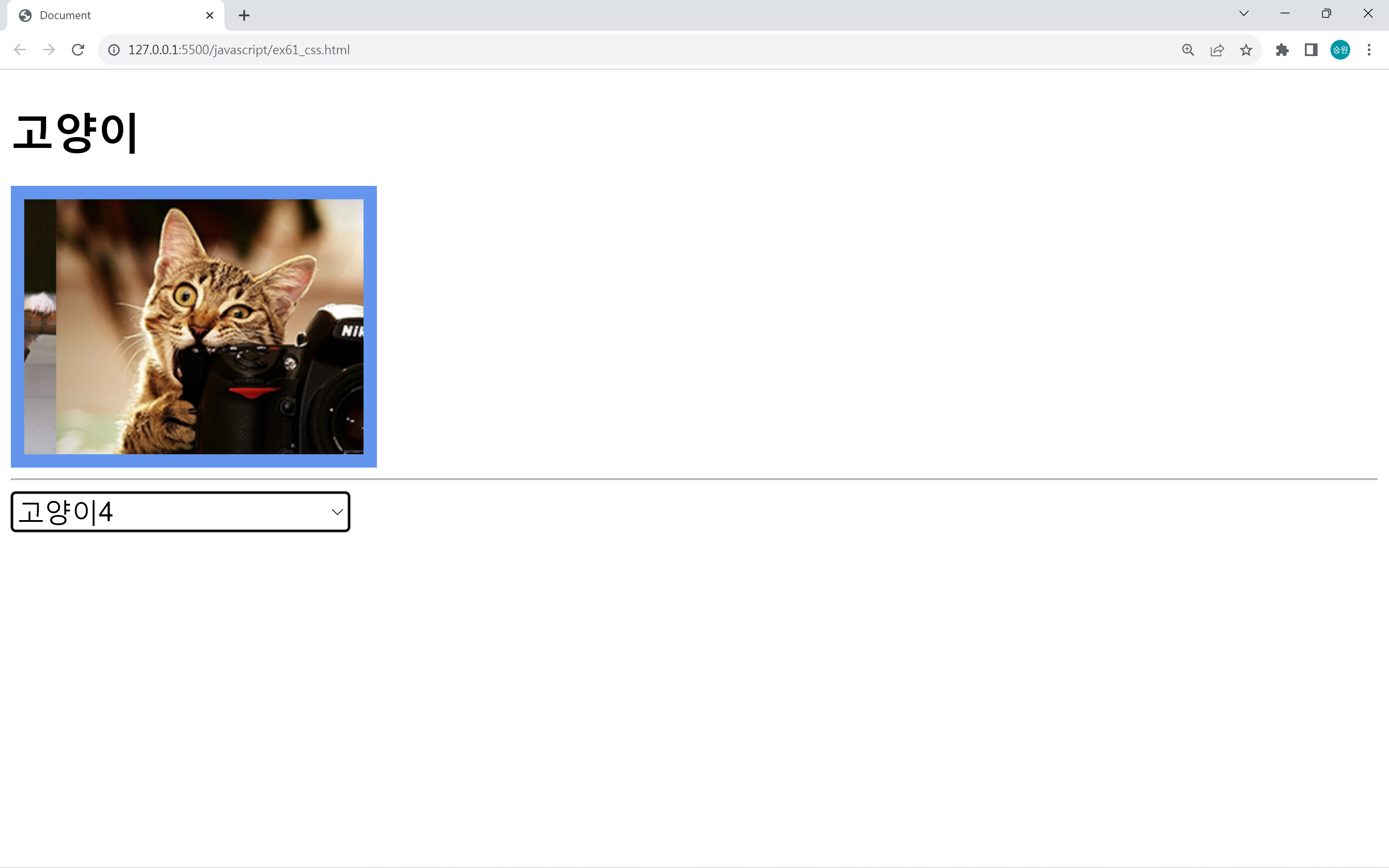
항목을 선택하거나 키보드 방향키를 입력하면 사진이 자연스럽게 다음으로 넘어간다.
배경 이미지 조작
하트
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<style>
#heart {
border: 1px solid black;
width: 300px;
height: 300px;
background-image: url(../asset/images/heart.png);
background-repeat: no-repeat;
}
</style>
</head>
<body>
<h1>배경 이미지 조작</h1>
<div id="heart"></div>
<hr>
<input type="button" value="두근 두근" id="btn1">
<script>
let x = 0;
document.getElementById('btn1').onclick = function() {
x -=300;
if (x < -600) {
x = 0;
}
document.getElementById('heart').style.backgroundPosition = x + 'px 0px';
}
</script>
</body>
</html>
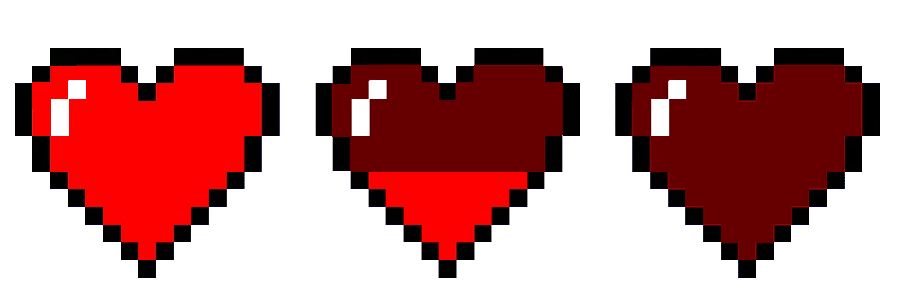
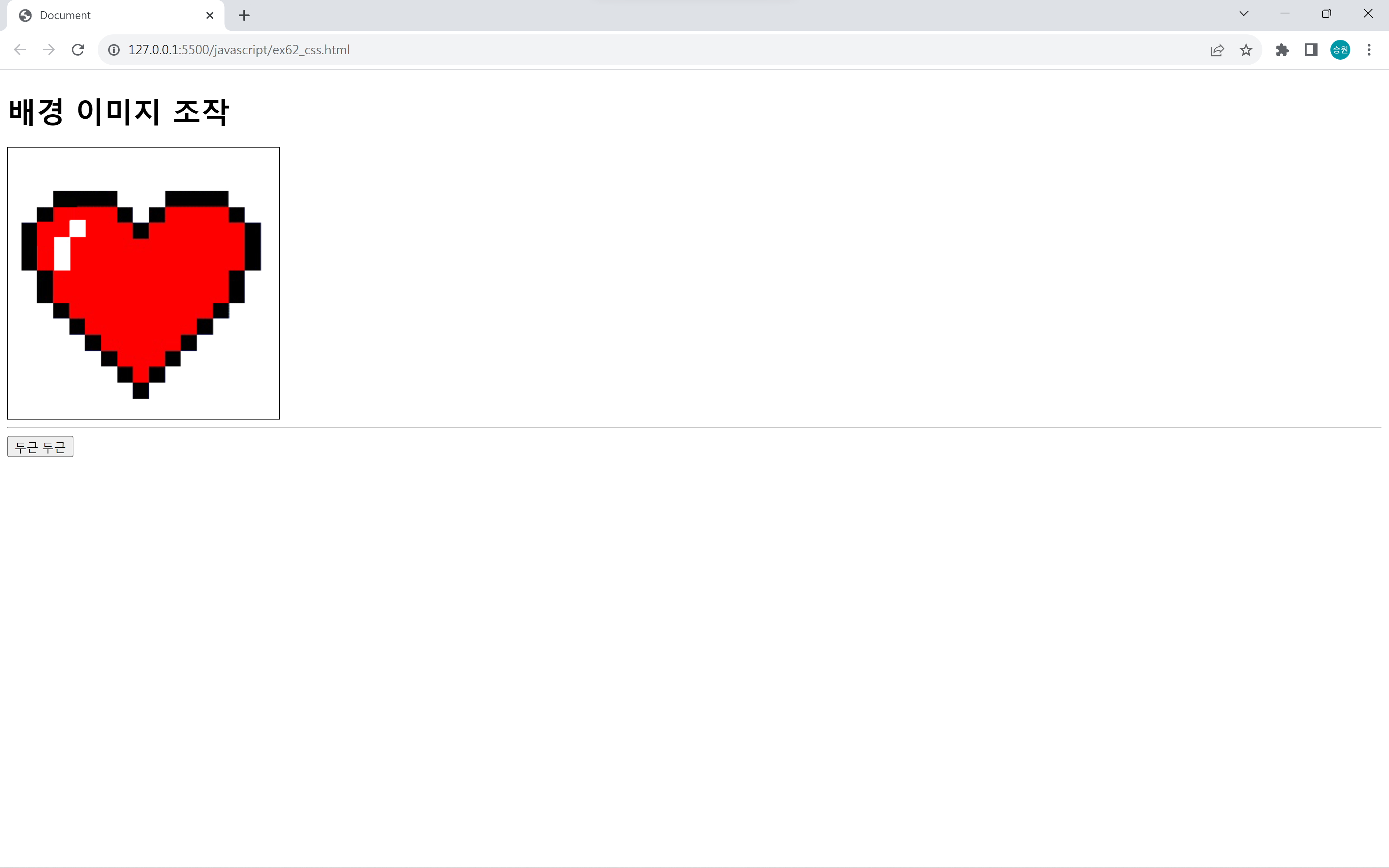
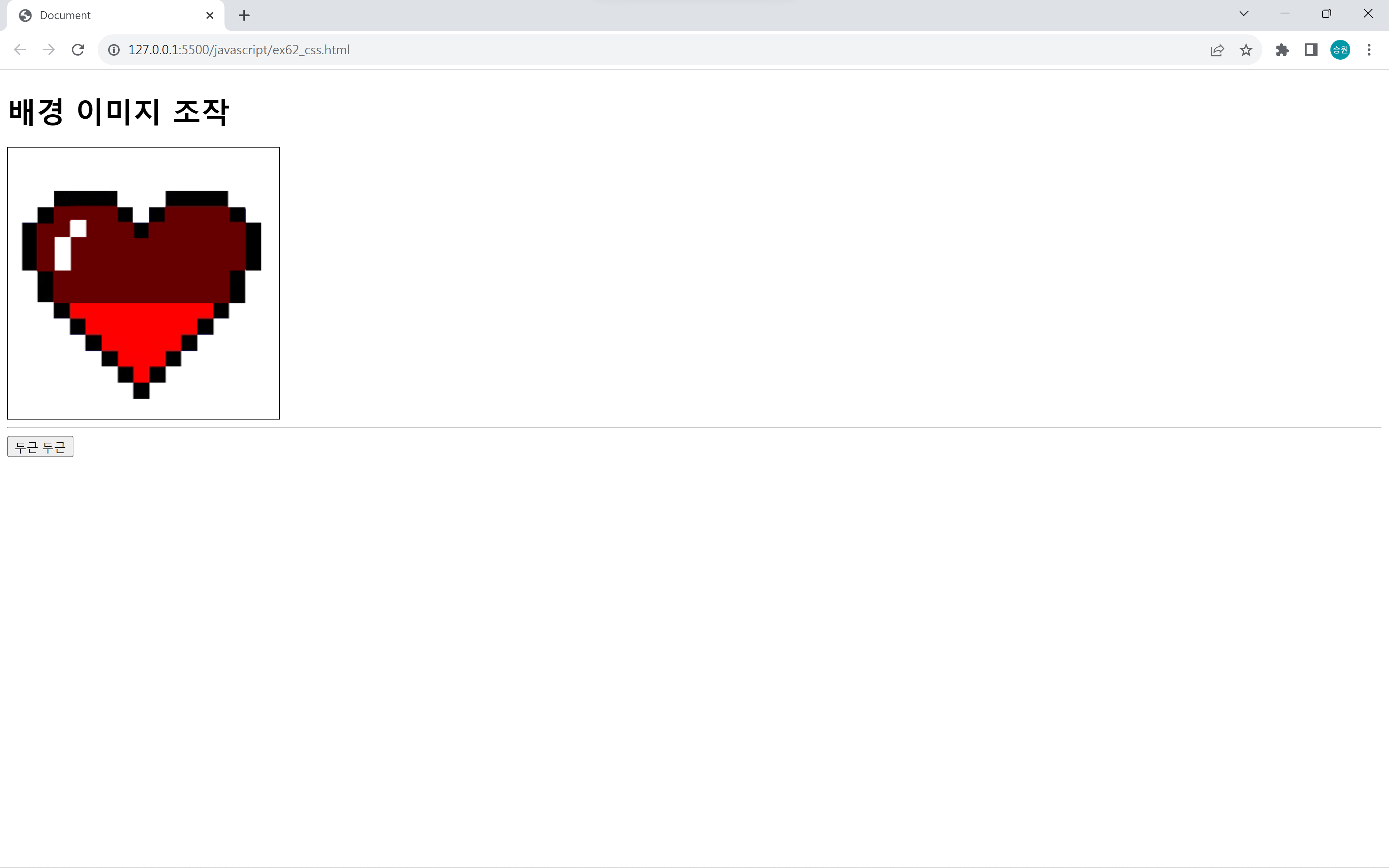
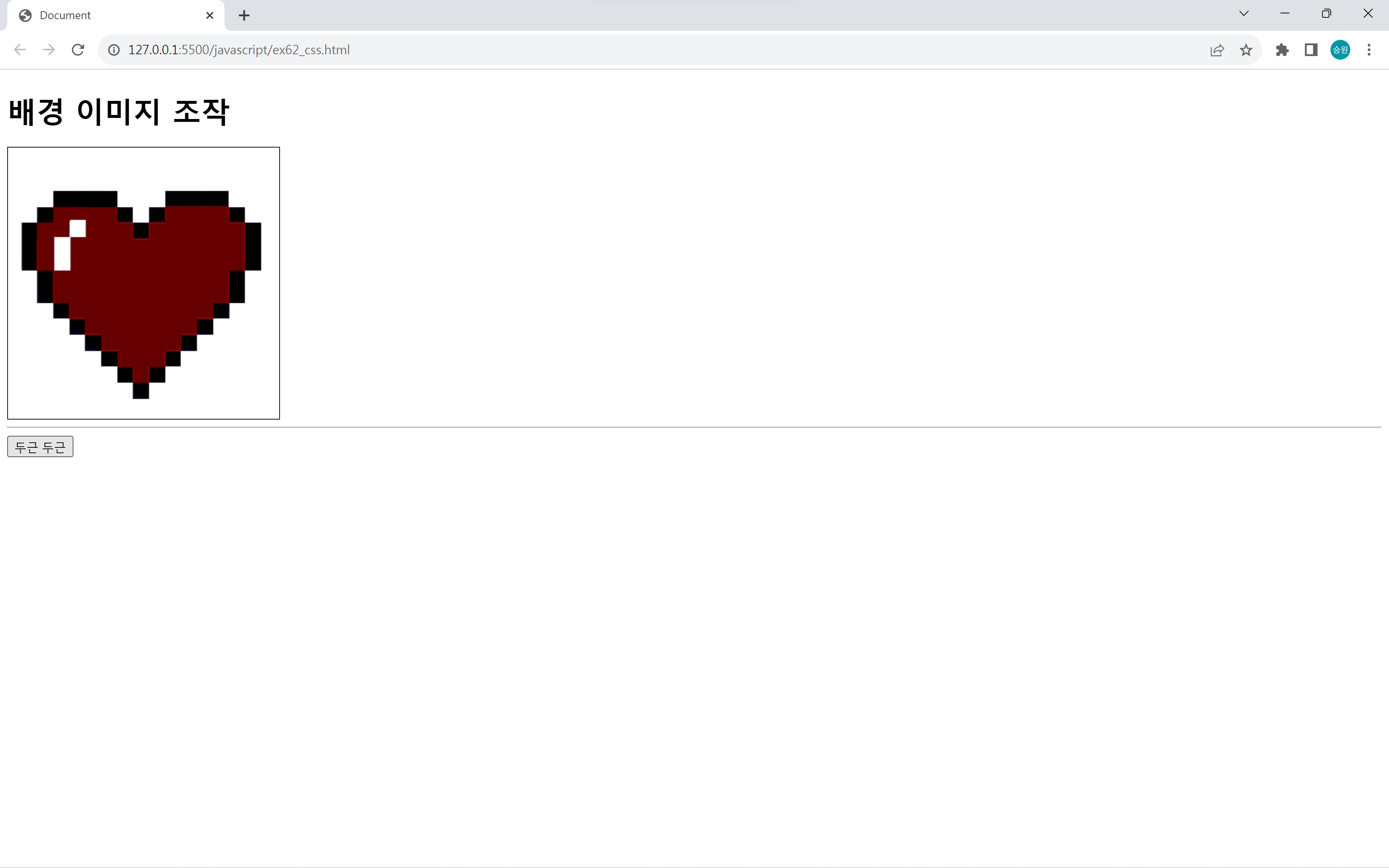
움직일 거리를 담당할 변수 x를 만들었다.
버튼을 클릭하면 하트 이미지가 전환된다.
퓨마
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<style>
#puma {
border: 1px solid black;
width: 510px;
height: 256px;
background-image: url(../asset/images/puma.png);
background-repeat: no-repeat;
}
</style>
</head>
<body>
<h1>퓨마</h1>
<div id="puma"></div>
<script>
const puma = document.getElementById('puma');
let x = 0;
let y = 0;
let timer = 0;
puma.onclick = function() {
if (timer == 0) {
timer = setInterval(function() {
x -= 512;
if (x < -(512 * 3)) {
x = 0;
y -= 256;
if (y < -256) {
y = 0;
}
}
puma.style.backgroundPosition = `${x}px ${y}px`;
}, 100);
} else {
clearInterval(timer);
timer = 0;
}
};
</script>
</body>
</html>

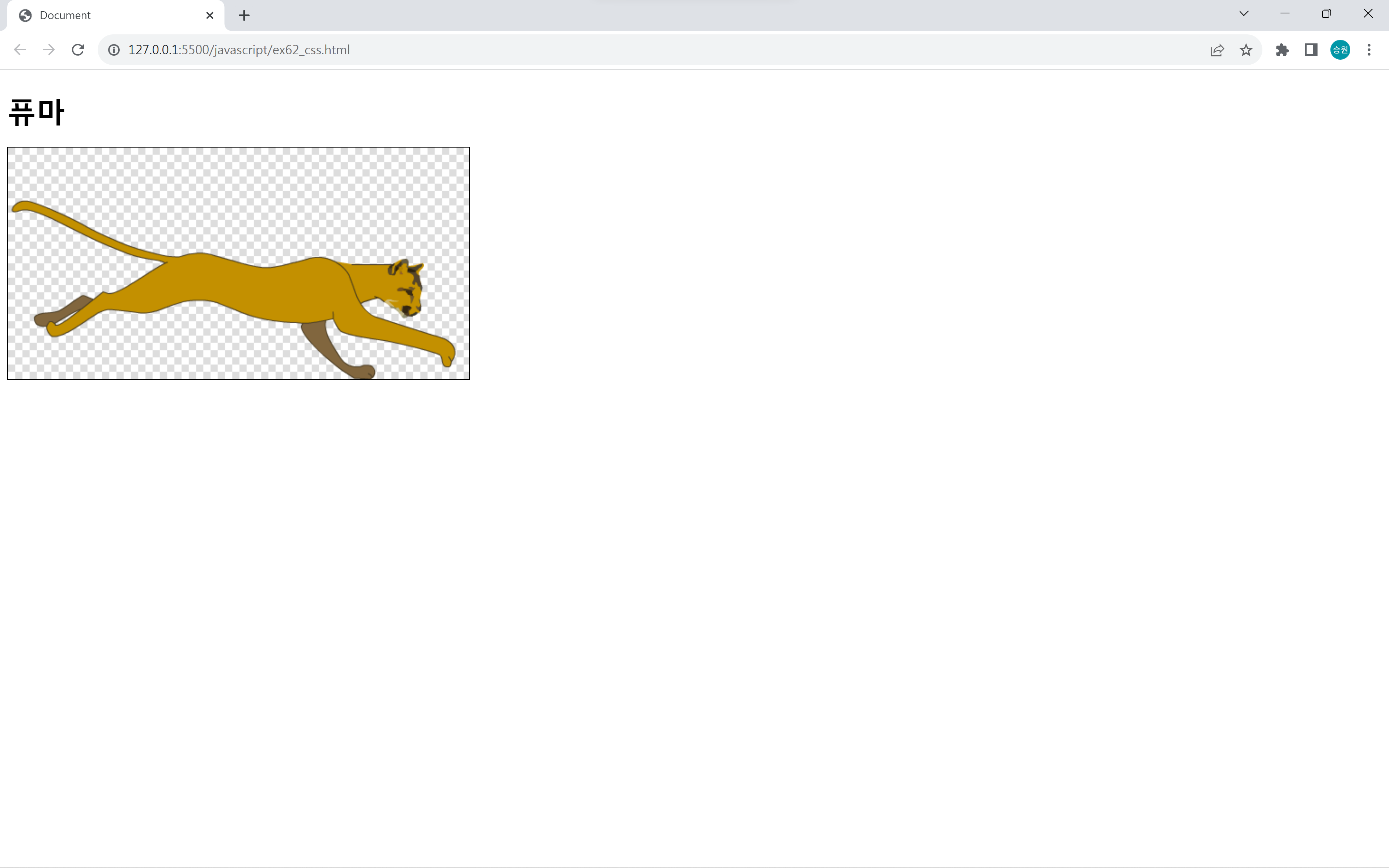
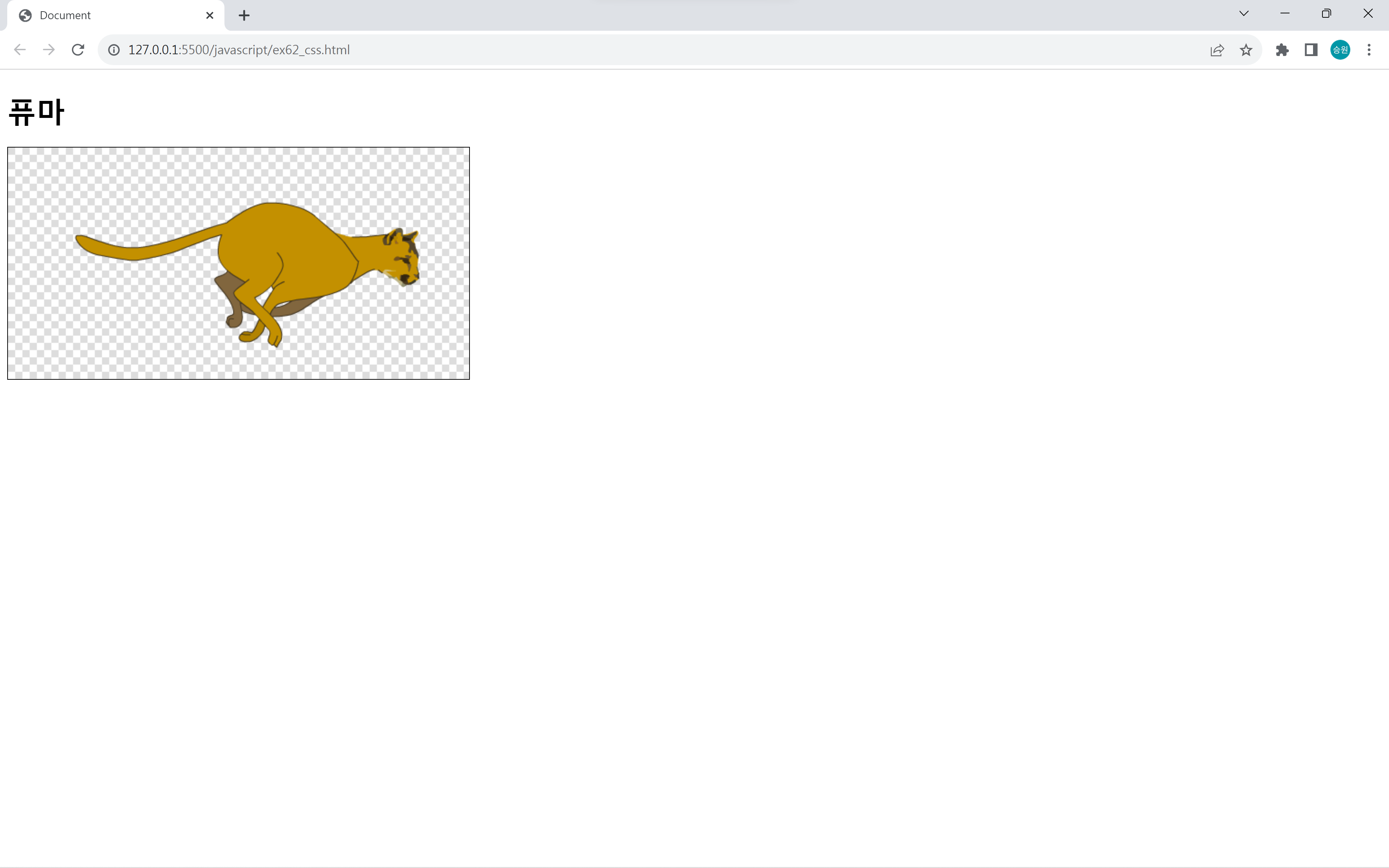
퓨마 이미지를 클릭하면 이미지가 순차적으로 전환하면서 퓨마가 뛰는 것처럼 보이게 된다.